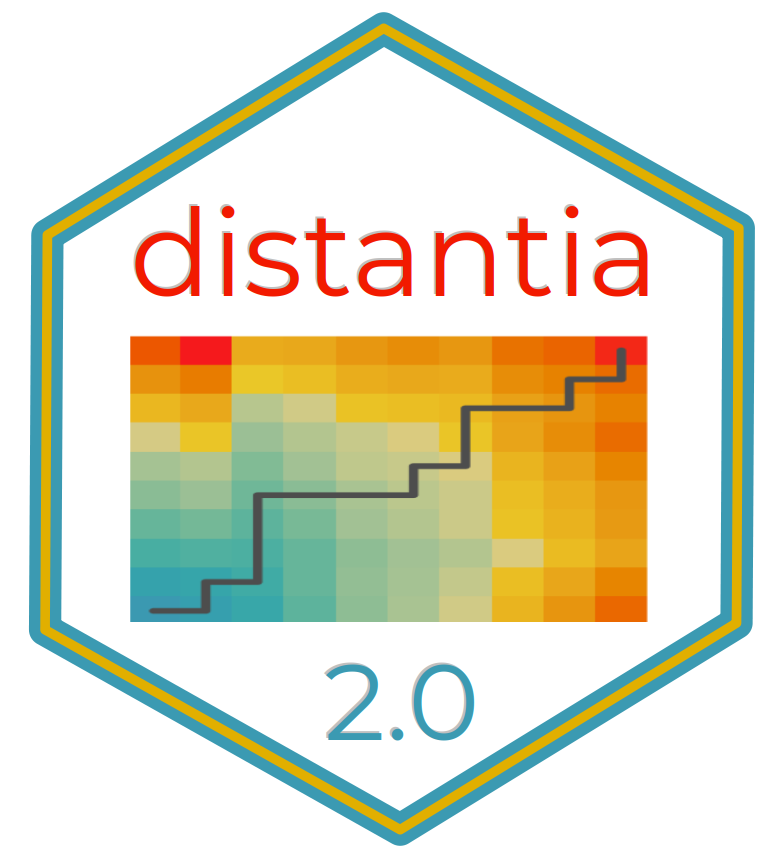
Convert Dissimilarity Analysis Data Frames to Spatial Dissimilarity Networks
Source:R/distantia_to_sf.R
distantia_to_sf.Rd
Given an sf data frame with the coordinates of a time series list transforms a data frame resulting from distantia()
to an sf data frame with lines connecting the time series locations. This can be used to visualize a geographic network of similarity/dissimilarity between locations. See example for further details.
Arguments
- df
(required, data frame) Output of
distantia()
ordistantia_aggregate()
. Default: NULL- xy
(required, sf POINT data frame) Sf data frame with the coordinates of the time series in argument 'df'. It must have a column with all time series names in
df$x
anddf$y
. See[eemian_cooordinaes]
example. Default: NULL
See also
Other dissimilarity_analysis:
distantia_aggregate()
,
distantia_boxplot()
,
distantia_cluster_hclust()
,
distantia_cluster_kmeans()
,
distantia_matrix()
,
distantia_plot()
,
distantia_stats()
,
distantia_time_shift()
,
momentum_boxplot()
,
momentum_stats()
,
momentum_to_wide()
Examples
#three time series
#climate and ndvi in Fagus sylvatica stands in Spain, Germany, and Sweden
data("fagus_dynamics")
data("fagus_coordinates")
#load as tsl
#center and scale with same parameters
tsl <- tsl_initialize(
x = fagus_dynamics,
name_column = "name",
time_column = "time"
) |>
tsl_transform(
f = f_scale_global
)
#example using distantia()
#--------------------------------------------------
distantia_df <- distantia(
tsl = tsl
)
#transform to sf
distantia_sf <- distantia::distantia_to_sf(
df = distantia_df,
xy = fagus_coordinates
)
#mapping with tmap
# library(tmap)
# tmap::tmap_mode("view")
#
# tmap::tm_shape(distantia_sf) +
# tmap::tm_lines(
# col = "psi",
# lwd = 3
# ) +
# tmap::tm_shape(fagus_coordinates) +
# tmap::tm_dots(size = 0.1, col = "gray50")
#example using momentum()
#--------------------------------------------------
importance_df <- momentum(
tsl = tsl
)
#transform to sf
importance_sf <- distantia::distantia_to_sf(
df = importance_df,
xy = fagus_coordinates
)
names(importance_sf)
#> [1] "edge_name" "y"
#> [3] "x" "psi"
#> [5] "most_similar" "most_dissimilar"
#> [7] "importance__evi" "importance__rainfall"
#> [9] "importance__temperature" "psi_only_with__evi"
#> [11] "psi_only_with__rainfall" "psi_only_with__temperature"
#> [13] "psi_without__evi" "psi_without__rainfall"
#> [15] "psi_without__temperature" "id.x"
#> [17] "id.y" "geometry"
# #map contribution to dissimilarity of evi
# #negative: contributes to similarity
# #positive: contributes to dissimilarity
# tmap::tm_shape(importance_sf) +
# tmap::tm_lines(
# col = "importance__evi",
# lwd = 3,
# midpoint = NA
# ) +
# tmap::tm_shape(fagus_coordinates) +
# tmap::tm_dots(size = 0.1, col = "gray50")
#
# #map variables making sites more similar
# tmap::tm_shape(importance_sf) +
# tmap::tm_lines(
# col = "most_similar",
# lwd = 3
# ) +
# tmap::tm_shape(fagus_coordinates) +
# tmap::tm_dots(size = 0.1, col = "gray50")
#
# #map variables making sites less similar
# tmap::tm_shape(importance_sf) +
# tmap::tm_lines(
# col = "most_dissimilar",
# lwd = 3
# ) +
# tmap::tm_shape(fagus_coordinates) +
# tmap::tm_dots(size = 0.1, col = "gray50")